Platform Web Service
Overview
The Platform web service provides methods for retrieving projects and their answer files from Intelledox. It also handles user authentication and maintenance, allowing user synchronisation with a third party system.
This service exposes a number of functions, or ‘methods’. These methods must be executed as an authenticated Intelledox user, and therefore the ‘Login’ method must always be called first. This creates a Session ID that is then used for subsequent calls to other methods.
Answer Files
Intelledox Answer Files are referred to often throughout this chapter. An Answer File is an XML data structure that contains the data captured by an Intelledox web form or document project. This data may include user responses to questions, data from external systems, and other content.
Intelledox uses Answer Files to save and pre-populate ‘In Progress’ web forms. Designers can control how specific responses are stored in the Answer File by setting Answer Labels in Designer. These labels become easy to read and write in an Answer File XML structure, as they are treated as simple ‘name/value’ pairs.
There are three types of Answer Files referred to:
- Default Answer Files – these have no data or responses apart from default values.
- Saved Answer Files – these are completed responses saved by the user in Produce after completing a web form.
- In Progress Answer Files – these are partially completed responses, either saved programmatically to pre-populate a web form, or saved part way through a web form by the end user.
GetAnswerFile Method
The GetAnswerFile method creates a default Answer File for any project, and returns it as XML data that can be manipulated and passed back to Intelledox to generate documents or pre-populate a web form. The default Answer File will contain default values where available for the project’s questions. Refer to Answer Files above for more information about each type of Answer File.
Using this method avoids the need to maintain ‘hard-coded’ answer files that become outdated when design changes are made to the project.
Often this method is used to ‘splice’ custom XML data into the Answer File before it is saved back to Intelledox, which is used to pre-populate a web form in Produce. This concept is described in the Pre-populating Web Forms section below.
The GetAnswerFile method requires a single parameter ‘templateGroupID’ which is an Integer value representing a project that has been published to a folder and can be accessed by authorised users.
Note
The templateGroupID is different to the ID of the project itself, as each project may be published to multiple folders with different publish options. The templateGroupID is used to identify a specific published instance of a project.
Also note that this method requires the Integer templateGroupID value and not the equivalent GUID value as in other methods.
The templateGroupID value can be sourced programmatically using the GetPublishedProjects method, which is described below:
GetAnswerFile Code Sample
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Xml;
namespace GetAnswerFile
{
class Program
{
static void Main(string[] args)
{
XmlDocument answerFileXML = new XmlDocument();
IxPlatformService.platformSoapClient IXPlatform = new IxPlatformService.platformSoapClient();
//tempalteGroupID must be sourced from the getPublishedProjects Method or from the Intelledox Database.
int templateGroupID = 1058;
//Call the Login web method, with the username and password.
var loginResult = new IxPlatformService.LoginResult();
try
{
loginResult = IXPlatform.Login("admin", "admin");
}
catch (Exception ex)
{
throw new ApplicationException("Login failed", ex);
}
//Add Session GUID information into the web service header
var sessionHeader = new IxPlatformService.SessionHeader();
sessionHeader.SessionId = loginResult.SessionID;
//Call web service and return answer file
answerFileXML.LoadXml(IXPlatform.GetAnswerFile(sessionHeader, templateGroupID));
if (answerFileXML.DocumentElement.SelectNodes("AnswerLabels/Label") != null)
{
foreach (XmlNode labelElement in answerFileXML.DocumentElement.SelectNodes("AnswerLabels/Label"))
{
labelElement.InnerText = "Test";
}
}
}
}
}
GetAnswerFiles Method
While the GetAnswerFile method retrieves a default Answer File for a single project, the GetAnswerFiles method retrieves an array of all saved Answer Files, which may include a number of different projects.
The Answer Files retrieved by this method have been saved by the end user from the Finish Page, after completing a web form in Produce. Refer to Answer Files above for more information about each type of Answer File.
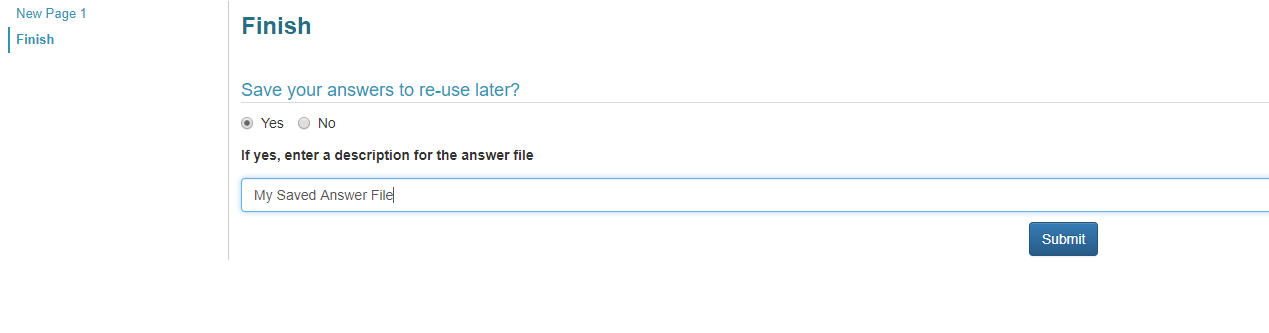
The GetAnswerFiles method returns an array of saved Answer Files for a particular user, based on the user specified in the Login method. This method is useful for creating a custom Intelledox interface or portal that enables users to retrieve saved Answer Files.
GetAnswerFiles Code Sample
The code below uses the GetAnswerFiles method to retrieve an array of answer file objects.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Xml;
namespace GetAnswerFiles
{
class Program
{
static void Main(string[] args)
{
var answerFiles = new List<IxPlatformService.AnswerFile>();
IxPlatformService.platformSoapClient IXPlatform = new IxPlatformService.platformSoapClient();
//Call the Login web method, with the username and password.
var loginResult = new IxPlatformService.LoginResult();
try
{
loginResult = IXPlatform.Login("admin", "admin");
}
catch (Exception ex)
{
throw new ApplicationException("Login failed", ex);
}
//Add Session GUID information into the web service header
var sessionHeader = new IxPlatformService.SessionHeader();
sessionHeader.SessionId = loginResult.SessionID;
//Call web service and return answer files
answerFiles = IXPlatform.GetAnswerFiles(sessionHeader).ToList();
}
}
}
Note
The GetAnswerFiles Method returns a list of available Answer Files but not the actual data, which is potentially quite large. To retrieve an Answer File’s actual data, a subsequent call to the getUserAnswerFile method is required, as described below.
GetInProgressAnswerFiles Method
In Progress Answer Files are those saved by the end user during the web form via the Save button, as depicted below. Refer to Answer Files above for more information about each type of Answer File.
The GetInProgressAnswerFiles method returns an array of in progress Answer Files for a particular user, based on the user specified in the Login method. This method is useful for creating a custom Intelledox interface or portal which allows users to return to partially completed web forms.
GetInProgressAnswerFiles Code Sample
The code below uses the GetAnswerFiles method to retrieve an array of answer file objects.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Xml;
namespace GetInProgressAnswerFiles
{
class Program
{
static void Main(string[] args)
{
var answerFiles = new List<IxPlatformService.AnswerFile>();
IxPlatformService.platformSoapClient IXPlatform = new IxPlatformService.platformSoapClient();
//Call the Login web method, with the username and password.
var loginResult = new IxPlatformService.LoginResult();
try
{
loginResult = IXPlatform.Login("admin", "admin");
}
catch (Exception ex)
{
throw new ApplicationException("Login failed", ex);
}
//Add Session GUID information into the web service header
var sessionHeader = new IxPlatformService.SessionHeader();
sessionHeader.SessionId = loginResult.SessionID;
//Call web service and return in-progress answer files
answerFiles = IXPlatform.GetInProgressAnswerFiles(sessionHeader).ToList();
}
}
}
Note
The GetInProgressAnswerFilesMethod returns a list of available answer files but not the actual ‘answerstring’ which is potentially quite large. This requires a subsequent getUserAnswerFile call described below.
GetPublishedProjects Method
The GetPublishedProjects method will return an array of projects that can be run by a particular user. The information can be used to create hyperlinks to projects, and is useful for creating a custom Intelledox interface or portal that allows users to launch Intelledox projects.
The method can be used to determine if a user has access to a particular project and return its ID or GUID for use with subsequent web service calls. The actual projects returned are those belonging to the user specified in the login call.
GetPublishedProjects Code Sample
The sample below gets a user’s published projects and iterates through them in their published folders.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace GetPublishedProjects
{
class Program
{
static void Main(string[] args)
{
var projList = new IxPlatformService.PublishedProjectList();
IxPlatformService.platformSoapClient IXPlatform = new IxPlatformService.platformSoapClient();
//Call the Login web method, with the username and password.
var loginResult = new IxPlatformService.LoginResult();
try
{
loginResult = IXPlatform.Login("admin", "admin");
}
catch (Exception ex)
{
throw new ApplicationException("Login failed", ex);
}
//Add Session GUID information into the web service header
var sessionHeader = new IxPlatformService.SessionHeader();
sessionHeader.SessionId = loginResult.SessionID;
projList = IXPlatform.GetPublishedProjects(sessionHeader);
if (projList != null)
{
foreach (IxPlatformService.Folder folder in projList.Folders)
{
var projects = new List<IxPlatformService.Project>();
//Discard folders for this sample, could be used to group the projects
foreach (IxPlatformService.Project project in folder.Projects)
{
projects.Add(project);
}
}
}
}
}
}
GetUserAnswerFile Method
The GetUserAnswerFile method will retrieve the properties for a particular AnswerFile saved by a user in a Produce web form. The answerFile requested must belong to the user specified in the login call.
The GetAnswerFile method requires a single input parameter, the answerFileId, which is an integer value representing a particular answerFile.
The ID value can be sourced programmatically using the getAnswerFiles and GetInProgressAnswerFiles methods described above or by running the following query on the Intelledox Database Directly.
SELECT u.Username, af.AnswerFile_ID, af.Description
FROM dbo.Answer_File AS af INNER JOIN
dbo.Intelledox_User AS u ON af.User_Guid = u.User_Guid
WHERE (u.Username = 'admin')
GetUserAnswerFile Code Sample
The code below uses the GetUserAnswerFile method to retrieve and begin to manipulate an answer file.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Xml;
namespace GetUserAnswerFile
{
class Program
{
static void Main(string[] args)
{
XmlDocument answerFileXML = new XmlDocument();
IxPlatformService.platformSoapClient IXPlatform = new IxPlatformService.platformSoapClient();
//AnswerrFileID must be sourced from the GetAnswerFiles/GetInprogress Method or from the Intelledox Database.
int answerFileID = 1058;
//Call the Login web method, with the username and password.
var loginResult = new IxPlatformService.LoginResult();
try
{
loginResult = IXPlatform.Login("admin", "admin");
}
catch (Exception ex)
{
throw new ApplicationException("Login failed", ex);
}
//Add Session GUID information into the web service header
var sessionHeader = new IxPlatformService.SessionHeader();
sessionHeader.SessionId = loginResult.SessionID;
//Call web service and return answer file
answerFileXML.LoadXml(IXPlatform.GetUserAnswerFile(sessionHeader, answerFileID).AnswerString);
//Access answerLabels
if (answerFileXML.DocumentElement.SelectNodes("AnswerLabels/Label") != null)
{
foreach (XmlNode labelElement in answerFileXML.DocumentElement.SelectNodes("AnswerLabels/Label"))
{
labelElement.InnerText = "Test";
}
}
}
}
}
Login Method
The login method is to authenticate a user to the platform web service. No other method can be called without a successfully building a session header via this method.
The credentials passed to the method when using the login service, must match the authentication type used by the Intelledox method.
The GetAnswerFile method requires a username and password parameters, passed as strings.
Login Code Sample
The samples below create a platform web service session header for both forms and windows authentication.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Login
{
class Program
{
static void Main(string[] args)
{
//Log in via Forms Authentication
var formsAuthResult = LoginFormsAuth("admin","admin");
//Log in via Windows Authentication
var windowsAuthResult = LoginWindowsAuth();
}
public static bool LoginFormsAuth(string username, string password)
{
bool result = false;
//Uses Intelledox Platform web service to log in via Forms Authentication and return a valid Session GUID.
IxPlatformService.platformSoapClient IXPlatform = new IxPlatformService.platformSoapClient();
//Call the Login web method, with the username and password.
var loginResult = new IxPlatformService.LoginResult();
try
{
loginResult = IXPlatform.Login(username, password);
}
catch (Exception ex)
{
throw new ApplicationException("Login failed", ex);
}
//Add Session GUID information into the web service header
var sessionHeader = new IxPlatformService.SessionHeader();
sessionHeader.SessionId = loginResult.SessionID;
if (sessionHeader.SessionId != Guid.Empty)
{
result = true;
}
return result;
}
public static bool LoginWindowsAuth()
{
//Uses Intelledox Platform web service to log in via Windows Authentication
//and return a valid Session GUID. Note: This will work only for Intelledox
//instances configured specifically for Windows Authentication.
bool result = false;
//Get the current Windows user
var userId = System.Security.Principal.WindowsIdentity.GetCurrent().Name;
if (userId.Contains(@"\"))
{
//strip domain from user name
userId = userId.Substring(userId.IndexOf(@"\") + 1);
}
//Uses Intelledox Platform web service to log in via Windows Authentication and return a valid Session GUID.
IxPlatformService.platformSoapClient IXPlatform = new IxPlatformService.platformSoapClient();
//Call the Login web method, with the username and password.
var loginResult = new IxPlatformService.LoginResult();
try
{
loginResult = IXPlatform.Login(userId, string.Empty);
}
catch (Exception ex)
{
throw new ApplicationException("Login failed", ex);
}
//Add Session GUID information into the web service header
var sessionHeader = new IxPlatformService.SessionHeader();
sessionHeader.SessionId = loginResult.SessionID;
if (sessionHeader.SessionId != Guid.Empty)
{
result = true;
}
return result;
}
}
}
SaveProgress Method
The SaveProgress method is used to save an Answer File to a user’s profile, usually for the purpose of launching that file in a user’s browser. After a successful call, specifying the sessionId as part of the Produce URL will automatically launch the saved Answer File in the user’s browser.
SaveProgress Code Sample
The sample below saves an answerfile and launches it in the user’s browser.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
using System.Xml;
namespace SaveProgress
{
class Program
{
static void Main(string[] args)
{
XmlDocument answerFileXML = new XmlDocument();
IxPlatformService.platformSoapClient IXPlatform = new IxPlatformService.platformSoapClient();
int userID = 1;
string answerFile = File.ReadAllText("C:\\temp\\answerFile.xml");
answerFileXML.LoadXml(answerFile);
//Configure the UserInfo node to the current user.
answerFileXML.SelectSingleNode("//HeaderInfo/UserInfo").Attributes["UserId"].InnerText = userID.ToString();
//Call the Login web method, with the username and password.
var loginResult = new IxPlatformService.LoginResult();
try
{
loginResult = IXPlatform.Login("admin", "admin");
}
catch (Exception ex)
{
throw new ApplicationException("Login failed", ex);
}
//Add Session GUID information into the web service header
var sessionHeader = new IxPlatformService.SessionHeader();
sessionHeader.SessionId = loginResult.SessionID;
//Save the answer file using the Platform web service.
IXPlatform.SaveProgress(sessionHeader, answerFileXML.OuterXml);
//Generate a URL
string url = "http://server/intelledox/produce";
url += "?SessionId=" + sessionHeader.SessionId.ToString();
//To open the web form in Portal mode, uncoment the following line.
//url += "&Portal=1"
}
}
}
SyncUser Method
Launching a saved Answer File within a user’s browser is discussed more in the Pre-Populated Web Forms section below.
SyncUser Code Sample
The sample below creates an Intelledox user. If a user ID was specified within the call the user’s credentials will be updated.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace SyncUser
{
class Program
{
static void Main(string[] args)
{
IxPlatformService.platformSoapClient IXPlatform = new IxPlatformService.platformSoapClient();
//Groups user will be assigned to
var groups = new Guid[] { new Guid("4396FD90-F339-4443-B9BA-F8264FE6AE6F") };
var user = new IxPlatformService.User();
var userAddress = new IxPlatformService.Address();
userAddress.Email = "[email protected]";
userAddress.Organisation = "Intelledox";
userAddress.Address1 = "123 Fake St";
user.Username = "myuser";
user.Password = "mypassword";
user.AddressDetails = userAddress;
//Call the Login web method, with the username and password.
var loginResult = new IxPlatformService.LoginResult();
try
{
loginResult = IXPlatform.Login("admin", "admin");
}
catch (Exception ex)
{
throw new ApplicationException("Login failed", ex);
}
//Add Session GUID information into the web service header
var sessionHeader = new IxPlatformService.SessionHeader();
sessionHeader.SessionId = loginResult.SessionID;
//Call web service and return answer file
IXPlatform.SyncUser(sessionHeader, user, groups);
}
}
}
Pre populating an eForm with an XML string
One of the most common uses for the Platform web service is launching a pre-populated Produce web form from a third party application. For example, a workflow solution may launch a web form pre-populated with customer data. Often the Produce interface is re-skinned and the user may be unaware they are interacting with Intelledox.
In the example below, complete XML structure is ‘spliced’ into the Answer File. It can then be referred to in the web form and generated document. The DataSourceID is the ID of the data source in Manage.
Pre populating an eForm with an XML string Code Sample
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Xml;
namespace PrePopulatingEForm
{
class Program
{
static void Main(string[] args)
{
//id of answerfile to retreive
int templateGroupID = 1057;
string produceUrl = "http://intelledox/Intelledox/Produce/";
string dataSourceID = "55efd0d0-22f6-40dd-9a21-1f22e7846c8b";
IxPlatformService.platformSoapClient IXPlatform = new IxPlatformService.platformSoapClient();
string url = string.Empty;
var answerFileXML = new XmlDocument();
//Call the Login web method, with the username and password.
var loginResult = new IxPlatformService.LoginResult();
try
{
loginResult = IXPlatform.Login("admin", "admin");
}
catch (Exception ex)
{
throw new ApplicationException("Login failed", ex);
}
//Add Session GUID information into the web service header
var sessionHeader = new IxPlatformService.SessionHeader();
sessionHeader.SessionId = loginResult.SessionID;
//Load the blank Answer File
answerFileXML.LoadXml(IXPlatform.GetAnswerFile(sessionHeader, templateGroupID));
//Configure the UserInfo node to the current user.
answerFileXML.SelectSingleNode("//HeaderInfo/UserInfo").Attributes["UserId"].InnerText = loginResult.UserId.ToString();
//Create and add the provided Data Node
var providedDataElement = answerFileXML.CreateNode(XmlNodeType.Element, "ProvidedData", "");
var dataElement = answerFileXML.CreateElement("Data");
var providedDataIdAttribute = answerFileXML.CreateAttribute("Id");
var customXML = answerFileXML.CreateCDataSection("<spliceData>" +
"<field_01>John</field_01>" +
"<field_02>Doe</field_02>" +
"<field_03>True</field_03>" +
"<field_04></field_04>" +
"<field_05></field_05>" +
"</spliceData>");
providedDataIdAttribute.Value = dataSourceID;
dataElement.Attributes.Append(providedDataIdAttribute);
dataElement.AppendChild(customXML);
providedDataElement.AppendChild(dataElement);
//Add the new element to the XMLdocument
var root = answerFileXML.DocumentElement;
root.AppendChild(providedDataElement);
//Pass the answerfile Back to Intelledox
IXPlatform.SaveProgress(sessionHeader, answerFileXML.OuterXml);
//Generate a URL
url = produceUrl + "?SessionId=" + loginResult.SessionID.ToString();
//To open the form in Portal mode, uncoment the following line.
//url += "&Portal=1"
//Open the URL in the browswer.
}
}
}
Updated about 6 years ago