GenerateDoc Web Service
Overview
The generateDoc service is for situations when document generation does not require end user input. Most commonly an XML structure is passed to the service and resulting documents are returned to the calling application or pushed to another system via an Action.
The service comprises of the generateWithData and Generate methods that are both designed to generate complete documents. The generateWithData method accepts a projectID and a custom XML data string whereas the generate method requires a complete Infiniti answerFile to generate a document.
The service endpoint is located here:
http://YourServer/Infiniti/Produce/Service/GenerateDoc.asmx
GenerateWithData Method
The GenerateWithData method accepts XML data produced by an external source and generates one or more complete documents. The resulting documents can be returned to the calling application or passed to other systems using an action. The results such as a success flags, URLs, a ticketID etc. can too be passed back to the calling application. Each message in passed back has a severity and description.
The method requires the following parameters.
Parameter | Description |
---|---|
Username | A username to authenticate to Intelledox as. |
Password | The password for the specified username, not required for windows authentication. |
ProjectGroupGuid | A GUID representing which published Infiniti project to generate. |
Data | An array containing one or more Provided Data objects. A provided data object is made of up the following parts: - Data – An XML string containing data produced by an external source - DataServiceGuid – A GUID representing the data source. For projects with that only use a single data source an empty GUID can be used here. Albeit possible to pass many different XML strings, generally only a single string is passed. |
Options | An object containing options that can be set to suit the situation, each is set to true or false. - LogGeneration – Store a record of the call within the Intelledox logs. - ReturnDocuments – Return the generated document to the calling application. Often delivery extensions are responsible for passing the document to another system and returning the document to a calling application is unnecessary network traffic. - RunProviders – Enables or disables action extensions configured to run for this project. |
GenerateWithData Code Sample
The sample below shows you how to Generate a Word or PDF Document from an XML String. In this example we are going to create a Console Application that passes a single XML structure to the generateWithData method and writes the first document returned by the service to the C:\Temp\
folder and iterates though any returned messages.
- Open Visual Studio and create a new Console Application project and give it a meaningful name. For this example, we will use ‘GenerateWithData’.
- To enable the project to access the Web services, a service reference will need to be added. In Visual Studio
Solution Explorer
, right-click the name of the project then clickAdd Service Reference
.
The Add Service Reference
dialog box appears.
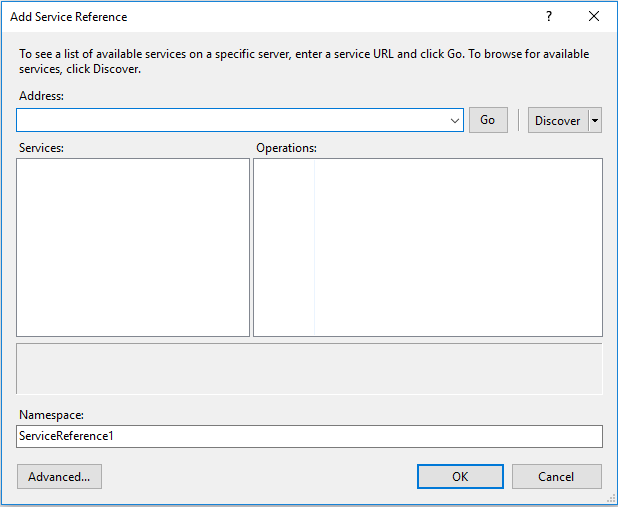
- In the
URL
box, enter the URL of Infiniti SOAP Web service to use. It should behttp://YourServer/Infiniti/Produce/Service/GenerateDoc.asmx
. - In the
Namespace
box, enter the namespace that you want to use for the reference. In this example we are going to useIxGenerateService
.
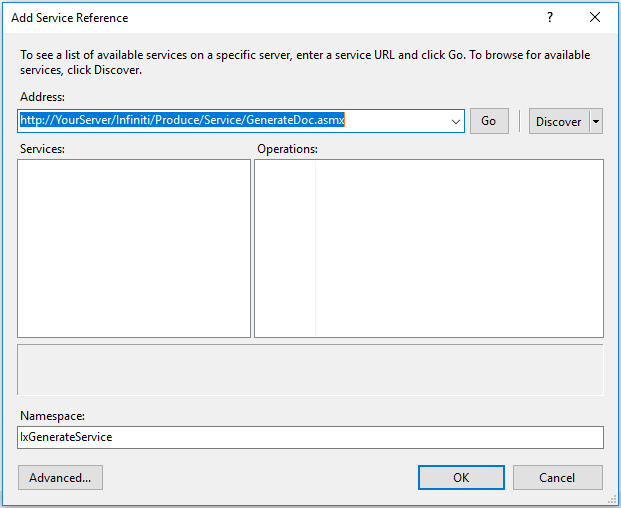
- Code your Console Application to perform as per sample code below.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace GenerateWithData
{
class Program
{
static void Main(string[] args)
{
IxGenerateService.GenerateDocSoapClient genService = new IxGenerateService.GenerateDocSoapClient();
string username = "admin";
string password = "admin";
Guid projectGroupGuid = new Guid("fcf24d3f-d15d-4b53-8a9f-615b1eb04262");
IxGenerateService.ProvidedData[] data = new IxGenerateService.ProvidedData[1];
IxGenerateService.ProvidedData externalData = new IxGenerateService.ProvidedData();
IxGenerateService.GeneratedDocument[] results;
IxGenerateService.GenerationOptions options = new IxGenerateService.GenerationOptions();
//Configure Options
options.LogGeneration = true;
options.ReturnDocuments = true;
options.RunProviders = true;
//Configure the Provided data object and add it to the array
externalData.DataServiceGuid = new Guid("55efd0d0-22f6-40dd-9a21-1f22e7846c8b");
externalData.Data = ("<spliceData>" +
"<field_01>John</field_01>" +
"<field_02>Doe</field_02>" +
"<field_03>True</field_03>" +
"<field_04></field_04>" +
"<field_05></field_05>" +
"</spliceData>");
data[0] = externalData;
//Call GenerateWithData and write the returned file to the c:\temp folder
results = genService.GenerateWithData(username, password, projectGroupGuid, data, options);
File.WriteAllBytes("c:\\temp\\" + results[0].FileName, results[0].BinaryFile);
//Iterate through any messages
foreach (IxGenerateService.Message m in results[0].Messages)
{
switch (m.Severity)
{
case IxGenerateService.MessageLevel.Info:
Console.WriteLine(m.Description);
break;
case IxGenerateService.MessageLevel.Warning:
Console.WriteLine(m.Description);
break;
case IxGenerateService.MessageLevel.Error:
Console.WriteLine(m.Description);
break;
}
}
}
}
}
- Replace
username
,password
,projectGroupGuid
,DataServiceGuid
, andData
with proper values from your environment. - Run the project, notice the generated document is written to
C:\Temp\
folder.
Generate Method
The generate service constructs a document from a complete answerFile. The answerFile can be built either entirely by the calling application or with the assistance of the platform service. After the document is generated it is returned to the calling application as base64 string represents a zip file that contains all generated document.
Unlike the platform service the generate service accepts login credentials as parameters rather than using a dedicated login method.
Generate Code Sample
The sample below constructs an answerFile containing a single customerID with the assistance of the platform service. The resulting zip file is saved in the C:\Temp\
directory.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
using System.Xml;
namespace Generate
{
class Program
{
static void Main(string[] args)
{
int templateGroupID = 1058; //id of answerfile to retreive
int CustomID = 10; //sampleId to include in answerFile
//*******************************************************************************************************
// --- Build an answer file with platform service, any technique can be used to build an answer file ---
//*******************************************************************************************************
IxPlatformService.platformSoapClient IXPlatform = new IxPlatformService.platformSoapClient();
XmlDocument answerFileXML = new XmlDocument();
//Call the Login web method, with the username and password.
var loginResult = new IxPlatformService.LoginResult();
try
{
loginResult = IXPlatform.Login("admin", "admin");
}
catch (Exception ex)
{
throw new ApplicationException("Login failed", ex);
}
//Add Session GUID information into the web service header
var sessionHeader = new IxPlatformService.SessionHeader();
sessionHeader.SessionId = loginResult.SessionID;
//Load the blank Answer File
answerFileXML.LoadXml(IXPlatform.GetAnswerFile(sessionHeader, templateGroupID));
//Set User Id information to the current user
answerFileXML.SelectSingleNode("//HeaderInfo/UserInfo").Attributes["UserId"].InnerText = loginResult.UserId.ToString();
//Include customID in AnswerFile
answerFileXML.DocumentElement.SelectSingleNode("AnswerLabels/Label[@name='CustomID']").InnerText = CustomID.ToString();
//***********************************************************************************************
// --- Pass the answerfile to the generate method ---
//***********************************************************************************************
IxGenerateService.GenerateDocSoapClient genService = new IxGenerateService.GenerateDocSoapClient();
var myDoc = genService.Generate("admin", "admin", answerFileXML.OuterXml);
File.WriteAllBytes("C:\\temp\\Generate.zip", myDoc);
}
}
}
Updated about 6 years ago